Interactive Notifications
Interactive Notifications are a great way to let the user interact with your app without having to open it.
Marigold supports sending to both iOS and Android to activate Interactive Notifications.
On iOS
On iOS, interactive notifications are configured via the UNNotificationSettings, UNNotificationCategory, UNNotificationAction classes, and their mutable counterparts.
On a UIUserNotificationCategory, an identifier
property is set, which a push payload should include as category
to activate this, plus any other relevant data.
On iOS, use the startEngine
overload and call startEngine:registerForPushNotifications:
with a NO
value. Then register with your own types and settings.
On Android
Actions can be pre-defined for push notifications using the addAction()
method. Actions are grouped by a "category" attribute that is sent as a part of the push bundle. When a notification arrives that contains a matching category attribute, the push data is injected into the action's intent and attached to the notification.
//This Intent must explicitly define an Activity, Service, or BroadcastReceiver class.
Intent actionIntent = new Intent(this, MyBroadcastReceiver.class);
NotificationConfig config = new NotificationConfig();
config.addAction(
"category", // Action Category
R.drawable.ic_action_reply, // Action Icon
"Reply", // Action Text
actionIntent, // Intent that is fired upon tap.
PendingIntent.FLAG_UPDATE_CURRENT // PendingIntent Flags
);
new Marigold().setNotificationConfig(config);
//This Intent must explicitly define an Activity, Service, or BroadcastReceiver class.
val actionIntent = Intent(this, MyBroadcastReceiver::class.java)
val config = NotificationConfig()
config.addAction(
"category", // Action Category
R.drawable.ic_action_reply, // Action Icon
"Reply", // Action Text
actionIntent, // Intent that is fired upon tap.
PendingIntent.FLAG_UPDATE_CURRENT // PendingIntent Flags
)
Marigold().setNotificationConfig(config)
To add more Actions to a category, call addAction()
for each action and set them all to the same category.
RemoteInput
NotificationConfig#addAction(String, int, CharSequence, Intent, RemoteInput, int)
AddAction()
has an optional RemoteInput
parameter that allows for user input to be included in the data that is sent. See RemoteInput in the Android Documentation for more information, and see Direct Reply For how to handle inline replies.
Responding to Actions
Inside the bundle that is sent to the intent defined in addAction()
is a valued called "_nid"
. The hashcode of _nid
is the identifier for the notification in the system tray.
public void onReceive(Context context, Intent intent) {
String notificationId = intent.getStringExtra("_nid");
NotificationManager notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.cancel(notificationId.hashCode());
}
fun onReceive(context: Context, intent: Intent) {
val notificationId = intent.getStringExtra("_nid")
val notificationManager = context.getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager
notificationManager.cancel(notificationId.hashCode())
}
Another option is to use getActiveNotifications()
to get a reference to update the notification.
Sending a push
Once the category is defined and hard-coded by a developer, the key category
can be used in both the Marigold Dashboard, Notifications, and Messages APIs.
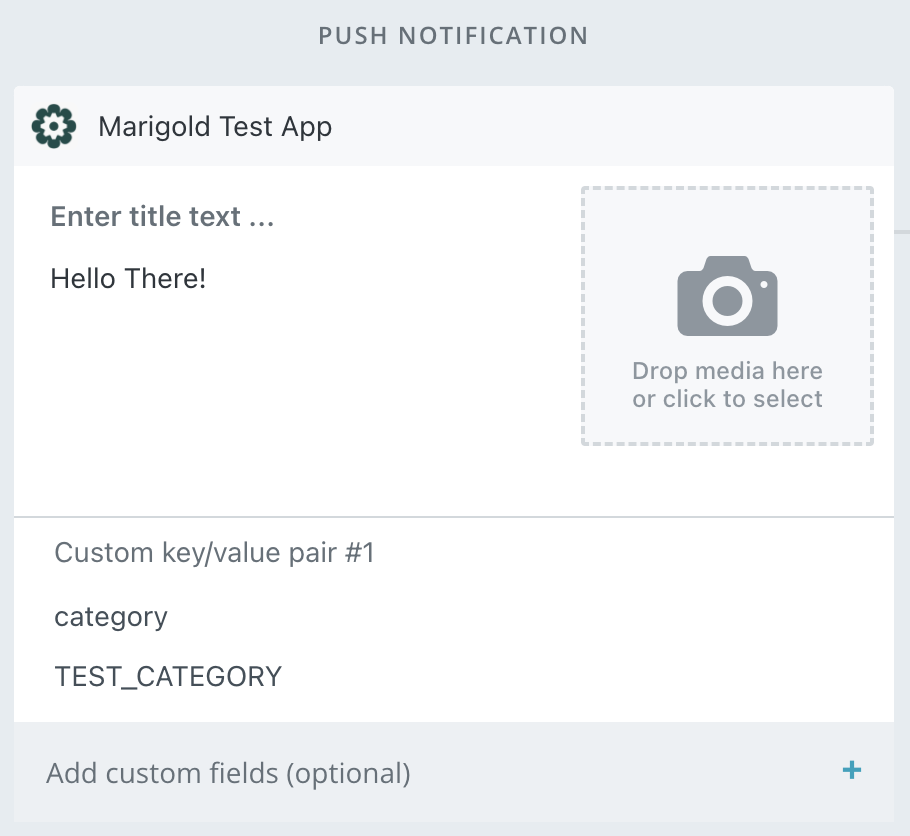
Fill in the 'category' key value to trigger your interactive notification.
API Example
Simply add the category
key and value to the payload.
curl -X POST -u BUNDLE_ID:API_KEY -H "Content-type: application/json" -H 'Accept: application/json' https://api.carnivalmobile.com/v3/notifications -d '{
"notification": {
"to": [{ "name": "tags", "criteria": ["tag1"]}, { "name": "custom.string.mykey", "criteria": ["tag2"]}],
"payload": {
"alert": "This is a push notification test",
"badge": 1,
"sound": "Default.caf",
"category": "TEST_CATEGORY",
"any_key": "any_value" //Arbitary keys/values.
}
}
}'
Updated 3 months ago